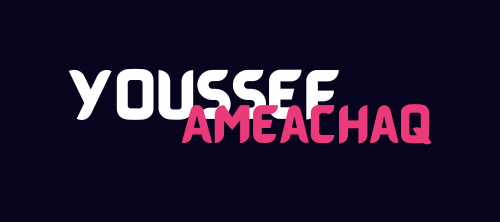
Introduction to event loop in Node.js
Introduction
In Node.js, the event loop is a fundamental concept that allows asynchronous operations to be performed efficiently. The event loop is responsible for handling I/O operations, timers, and callbacks in a non-blocking manner, making Node.js highly scalable and efficient.
Here’s a simplified explanation of how the event loop works in Node.js:
- The event loop starts by processing the main script that is executed when you run your Node.js application.
- As the script runs, any asynchronous operations, such as file I/O or network requests, are initiated. These operations are performed in the background, and Node.js does not block the execution of the main script.
- Once an asynchronous operation completes, it emits an event or invokes a callback function.
- The event loop picks up these events or callbacks and adds them to different queues based on their type. The two primary queues are the “Timers” queue and the “I/O” queue.
- The event loop continuously checks these queues for any pending events or callbacks.
- If a timer expires, the event loop moves the corresponding callback to the call stack, and it gets executed.
- If an I/O operation is complete, the event loop moves the corresponding callback to the call stack.
- The call stack executes the callbacks, and any synchronous code associated with them runs.
- Once the call stack is empty, the event loop continues to the next iteration, checking for new events or callbacks.
- The process repeats until there are no more events or callbacks to process, and the application exits or remains idle, waiting for new events to occur.
Example
Here’s an example that demonstrates the event loop in Node.js using some schemas and code:
- Main script:
console.log('Start');
setTimeout(() => {
console.log('Timeout callback');
}, 0);
setImmediate(() => {
console.log('Immediate callback');
});
console.log('End');
- Explanation:
- The script starts by printing ‘Start’ to the console.
setTimeout
is a timer function that schedules a callback function to be executed after a specified delay (in this case, 0 milliseconds).setImmediate
is a function that schedules a callback function to be executed in the next iteration of the event loop.- After scheduling the timers and immediate callbacks, ‘End’ is printed to the console.
- Output:
When you run the above script, the output will be as follows:
Start
End
Immediate callback
Timeout callback
- Explanation of the output:
- The ‘Start’ message is printed first.
- Then, the timers and immediate callbacks are scheduled in the event loop.
- ‘End’ is printed before any callbacks because it is synchronous code.
- Next, the immediate callback is executed, even though the timeout callback was scheduled first. This is because
setImmediate
callbacks are executed in the next iteration of the event loop. - Finally, the timeout callback is executed.
This example illustrates how the event loop processes different types of callbacks in the order defined by the event loop mechanism. Even though the timeout callback was scheduled first, the immediate callback is executed before it due to the event loop’s ordering.
The phases of the Event loop
The event loop in Node.js operates in different phases, each responsible for handling specific types of events and callbacks. The phases of the event loop are as follows:
-
Timers Phase: In this phase, the event loop checks if any timer callbacks are ready to be executed. This includes callbacks scheduled by
setTimeout()
andsetInterval()
. If a timer has expired, its callback is added to the callback queue to be executed. -
I/O Callbacks Phase: In this phase, the event loop handles I/O-related callbacks. These callbacks include those associated with network operations, file system operations, and other asynchronous I/O operations. When an I/O operation completes, its corresponding callback is added to the callback queue for execution.
-
Idle, Prepare Phase: This phase is used internally by the event loop and is generally not visible to developers.
-
Poll Phase: The poll phase is where most of the event loop’s work happens. It checks for new I/O events and executes their associated callbacks immediately. If there are no pending I/O callbacks, it may wait for events to arrive (blocking), effectively putting the event loop to sleep until new events occur.
-
Check Phase: In this phase, callbacks scheduled by
setImmediate()
are executed. These callbacks are executed immediately after the poll phase, regardless of whether the poll phase was blocked or not. -
Close Callbacks Phase: The close callbacks phase handles the callbacks for resources that have been closed, such as sockets or file handles. The associated callbacks are executed in this phase.
After executing the above six phases, the event loop starts a new iteration from the beginning, repeating the process to handle new events and callbacks. It continues to run as long as there are events and callbacks to process or until the application is explicitly terminated.
It’s important to note that the event loop in Node.js is designed to be single-threaded, meaning it can only process one event or callback at a time. However, its asynchronous and non-blocking nature allows it to efficiently handle multiple concurrent operations and deliver high performance.
process.nextTick()
The process.nextTick()
method in Node.js is used to schedule a callback function to be executed on the next iteration of the event loop. It allows you to defer the execution of a function until the current execution stack is empty, providing a way to prioritize certain operations and ensure they are executed as soon as possible.
Here’s an example that demonstrates the usage of process.nextTick()
:
console.log('Start');
process.nextTick(() => {
console.log('Next tick callback');
});
console.log('End');
When you run the above code, the output will be:
Start
End
Next tick callback
Explanation:
- The script starts by printing ‘Start’ to the console.
- The
process.nextTick()
function is called with a callback function as an argument. - The callback function is not executed immediately. Instead, it is scheduled to run on the next iteration of the event loop, after the current execution stack is empty.
- ‘End’ is printed to the console.
- On the next iteration of the event loop, the callback function passed to
process.nextTick()
is executed, printing ‘Next tick callback’ to the console.
The process.nextTick()
method is commonly used when you want to ensure that certain callbacks are executed before the event loop continues to process other callbacks and events. It is often used to defer execution of critical code, such as error handling or finalizing resources, to ensure they are not delayed by other pending operations.
Conclusion
The event loop in Node.js is crucial for managing concurrency and handling multiple requests efficiently. It ensures that resources are not wasted by waiting for I/O operations to complete, allowing Node.js to handle a large number of concurrent connections.